Installing Emscripten and Building Your First WASM Module
In order to learn WebAssembly, we can started by installing Emscripten, a popular toolchain for compiling C and C++ code to WASM. Emscripten is a free, open-source compiler toolchain that allows you to compile C and C++ code into WebAssembly modules. It provides a set of compilers, libraries, and tools that make it easy to create WASM modules for web applications.
In this article, we will explore how to install Emscripten and build your first WASM module.
Installing Emscripten
You can install Emscripten from source by cloning the repository from github
git clone https://github.com/emscripten-core/emsdk.git
After cloning the repository, you can install the SDK by running the following command:
cd emsdk
./emsdk install latest
to activate the SDK, you can run the following command:
./emsdk activate latest
source ./emsdk_env.sh
To make sure that the SDK is activated, you can run the following command:
emcc -v
The code above will show you the version of the emscripten that you have installed. However, if you want to make sure the emcc
command is working everytime you open a command line / terminal, you can use the script below inside the emsdk
directory:
echo "source $(pwd)/emsdk_env.sh" >> ~/.bashrc
if the SDK is activated, you will see the following output:
Setting up EMSDK environment (suppress these messages with EMSDK_QUIET=1)
Adding directories to PATH:
PATH += /home/dani/Documents/indiehacking/belajarwebassembly/emsdk/upstream/emscripten
Setting environment variables:
PATH = /home/dani/Documents/indiehacking/belajarwebassembly/emsdk/upstream/emscripten:/home/dani/Documents/indiehacking/belajarwebassembly/emsdk:/home/dani/.nvm/versions/node/v18.18.2/bin:/home/dani/miniconda3/bin:/home/dani/miniconda3/condabin:/tmp/.mount_cursorDL8Qch:/tmp/.mount_cursorDL8Qch/usr/sbin:/home/dani/.cargo/bin:/home/dani/.local/bin:/usr/local/sbin:/usr/local/bin:/usr/sbin:/usr/bin:/sbin:/bin:/usr/games:/usr/local/games:/snap/bin:/home/dani/Android/Sdk/emulator:/home/dani/Android/Sdk/platform-tools:/home/dani/minio-binaries/
EMSDK = /home/dani/Documents/indiehacking/belajarwebassembly/emsdk
EMSDK_NODE = /home/dani/Documents/indiehacking/belajarwebassembly/emsdk/node/18.20.3_64bit/bin/node
or if you using bash, you can add the following line to your .bashrc
or .zshrc
file:
export PATH=$(pwd)/emsdk:$PATH >> ~/.bashrc
so that every time you open a new terminal, the emsdk will be activated automatically.
Building your first WASM module
To build your first WASM module, you can create a simple hello world program in C:
#include <stdio.h>
int main() {
printf("Hello, World!\n");
return 0;
}
To compile this program to WASM, you can use the following command:
emcc hello.c
and you will get two files, a.out.wasm
and a.out.js
. The a.out.wasm
file is the WASM module, and the a.out.js
file is the glue code that allows you to run the WASM module in the browser.
you will see the directory structure like this:
a.out.wasm
a.out.js
hello.c
then you can create an HTML file to test the WASM module:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Hello WebAssembly</title>
</head>
<body>
<h1>Hello WebAssembly</h1>
<script src="a.out.js"></script>
</body>
</html>
To run the HTML file, you can use the following command:
emrun --browser chrome a.out.html
then your browser will open and you will see the following output in the console:
Hello, World!
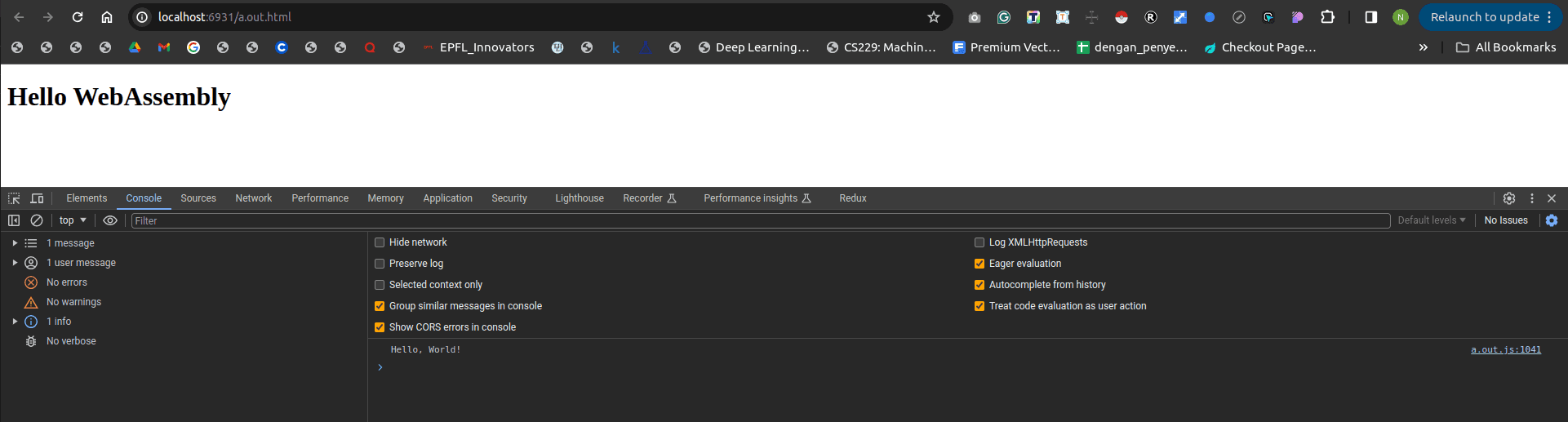
From the example above, we have successfully compiled C code into a WASM module and executed it directly in the browser.
References
- WebAssembly Crash Course - Basics [Video]. (2023). YouTube.